AlertNow Documentation
Learn how to integrate and use AlertNow to receive real-time alerts for your applications.
Installation
There are two ways to integrate AlertNow into your application: using our npm package or making direct API calls.
npm install alertnow
After installation, you'll need to initialize the client with your API key:
import { AlertNow } from 'alertnow';
// Initialize with your API key
const alertNow = new AlertNow('your-api-key');
Setting up Alert Rules
Before sending alerts, you need to configure your alert rules in the dashboard. This determines where your alerts will be sent and what conditions trigger them.
Alert Rules Configuration
Usage Examples
Once you've installed AlertNow and set up your alert rules, you can start sending alerts from your application. Here are some examples of how to use AlertNow in different scenarios.
Node.js Example
Here's how to send an alert using the Node.js client:
import { AlertNow } from 'alertnow';
// Initialize with your API key
const alertNow = new AlertNow('your-api-key');
try {
// Your application code
doSomethingRisky();
} catch (error) {
// Send an alert when an error occurs
alertNow.send(
"critical", // Alert level
"Runtime Error", // Alert title
error.message, // Alert message
{
userId: 1, // Additional data
errorStack: error.stack
}
);
}
// You can also send alerts proactively
function checkDatabaseConnection() {
const isConnected = pingDatabase();
if (!isConnected) {
alertNow.send(
"warning",
"Database Connection Issue",
"Unable to connect to the database",
{
databaseId: "main-db",
lastPingTime: new Date().toISOString()
}
);
}
}
REST API Example
If you're using the REST API directly, here's how to structure your request:
// Request Body
{
"level": "critical",
"title": "Runtime Error!",
"message": "Nil pointer at line 35",
"data": {
"userId": 1,
"serviceId": "auth-service",
"environment": "production"
}
}
Send this JSON payload to the AlertNow API endpoint:
curl -X POST https://api.alertnow.io/v1/alerts \
-H "Content-Type: application/json" \
-H "Authorization: Bearer your-api-key" \
-d '{
"level": "critical",
"title": "Runtime Error!",
"message": "Nil pointer at line 35",
"data": {
"userId": 1,
"serviceId": "auth-service",
"environment": "production"
}
}'
Receiving Alerts
AlertNow supports multiple notification channels to ensure you never miss an important alert. Here's how alerts appear in different channels:
Discord Integration
When an alert is triggered, it will appear in your configured Discord channel:
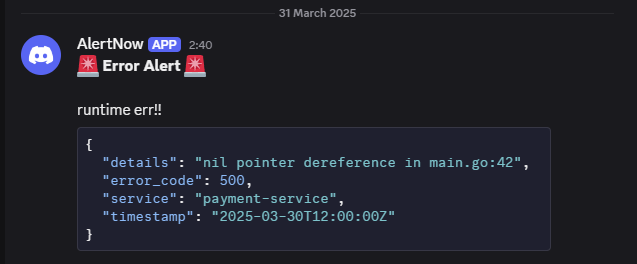
Example of an AlertNow notification in Discord
Discord notifications include:
- Alert level (color-coded)
- Alert title and message
- Additional data in an expandable section